1 min read
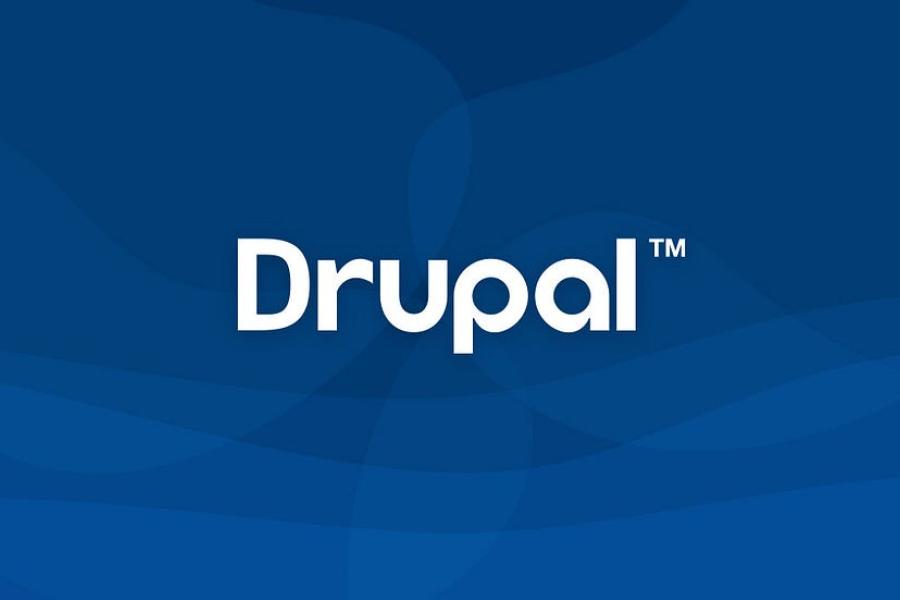
Services are the building blocks of a Drupal application. By creating custom services, you can extend Drupal in powerful ways, providing reusable functionality that can be injected into any part of the system.
**Defining a Custom Service**
Services are defined in `*.services.yml` files. Here's an example of defining a custom service:
```yaml
services:
mymodule.custom_service:
class: Drupal\mymodule\CustomService
arguments: ['@entity_type.manager']
Implementing the Custom Service
The custom service is implemented as a PHP class. This class can inject other services via its constructor:
namespace Drupal\mymodule;
use Drupal\Core\Entity\EntityTypeManagerInterface;
class CustomService {
protected $entityTypeManager;
public function __construct(EntityTypeManagerInterface $entity_type_manager) {
$this->entityTypeManager = $entity_type_manager;
}
public function doSomething() {
// Use the injected service to perform a task
$nodes = $this->entityTypeManager->getStorage('node')->loadMultiple();
// Additional logic here
}
}
Using the Custom Service
Once the service is defined, you can inject it into other classes, such as controllers or forms, and call its methods:
namespace Drupal\mymodule\Controller;
use Drupal\Core\Controller\ControllerBase;
use Symfony\Component\DependencyInjection\ContainerInterface;
use Drupal\mymodule\CustomService;
class MyController extends ControllerBase {
protected $customService;
public function __construct(CustomService $custom_service) {
$this->customService = $custom_service;
}
public static function create(ContainerInterface $container) {
return new static(
$container->get('mymodule.custom_service')
);
}
public function page() {
$this->customService->doSomething();
return [
'#markup' => $this->t('Custom service executed successfully.'),
];
}
}
Creating custom services in Drupal allows for clean, reusable, and testable code, improving overall application architecture.
Custom Services
,
Drupal
,
Backend Development
Share this article
- Log in to post comments